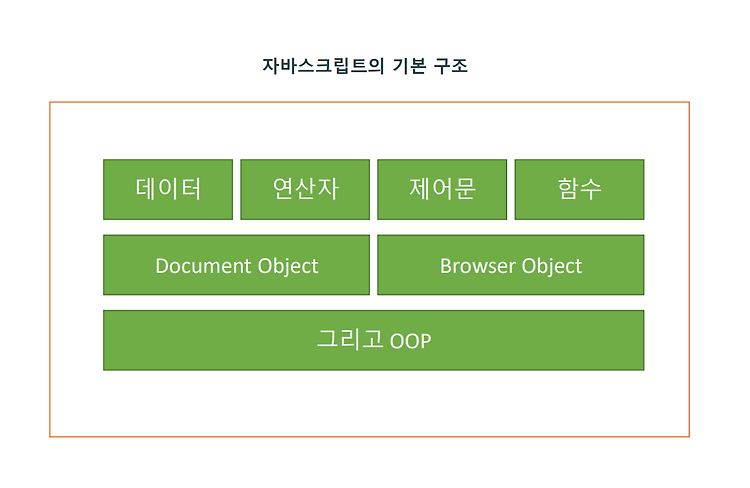
JavaScript 기초 1장
2022. 2. 21. 16:09
Language/JavaScript
오랜만에 글을 적습니다. 요즘에 깃을 많이 쓰려고 노력하다 보니 블로그에 소홀해졌네요 ~~ !! 다시 한번 제대로 적어보겠습니다. 자바 스크립트란? 프로토타입 기반 객체지향 언어 입니다. 스크립트 언어의 특성 상 플랫폼에 독립적이며 모든 웹 브라우저에서 동일한 실행 결과를 얻을수 있다. 무료이고 쉽고 자유롭게 사용할수 있습니다. 클라이언트에서만 실행되기에 정보를 서버에 보낼 필요 없이 처리할수 있다. 타입 체크가 철저하지 않습니다. 그런즉 변수들의 타입에 있어서 차이를 두지 않습니다. 자바스크립트 기반 프론트엔드 기술의 발전으로 근래, 순수 스크립트의 사용을 선호하게 되며 JAVA 보다 확장성이 뛰어난 언어입니다. 자바스크립트 개발자가 있을 만큼 사용방법이 다양하며, 반드시 익혀두어야합니다. JAVA 를..